This article is Part 3 of the series “Producing and Consuming OData in a Silverlight and Windows Phone 7 application.”.
To refresh your memory on what OData is:
The Open Data Protocol (OData) is simply an open web protocol for querying and updating data. It allows for the consumer to query the datasource (usually over HTTP) and retrieve the results in Atom, JSON or plain XML format, including pagination, ordering or filtering of the data.
To recap what we learned in the previous section:
- We began by creating the User Interface and setting up our bindings on our elements.
- We added a service reference pointing to our OData Data Service inside our project.
- Finally, we added code behind to sort and filter the data coming from our OData Data Source into our Silverlight 4 Application.
In this article, I am going to show you how to consume an OData Data Source using Windows Phone 7. Read the complete series of articles to have a deep understanding of OData and how you may use it in your own applications.
See the video tutorial
Download the source code for part 3 | Download the slides
Testing the OData Data Service before getting started…
Hopefully you have completed part 1 and 2 of the series, if you have not you may continue with this exercise by downloading the completed source code to Part 2 and continue. I would recommend watching the video to part 1 and part 2 in order to have a better understanding of where you can add OData to your own applications.
Go ahead and load the SLShowODataCompleted project inside of Visual Studio 2010, and look under Solution Explorer. Now right click CustomerService.svc and Select “View in Browser”.
If everything is up and running properly, then you should see the following screen (assuming you are using IE8).
Please note that any browser will work. I chose to use IE8 for demonstration purposes only.
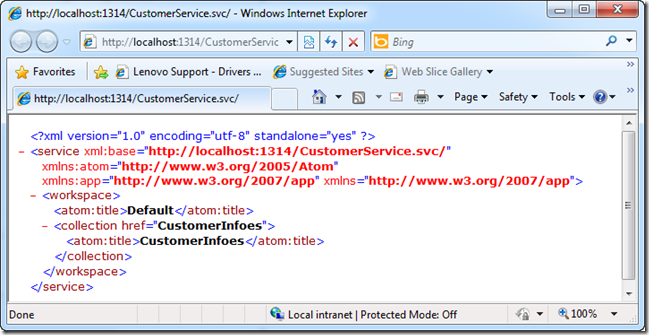
If you got the screen listed above then we know everything is working properly and can continue. If not download the completed solution and continue with the rest of the article.
At this point, leave this instance of Visual Studio running, we are going to use this OData Data Service in the “Getting Started” part. You may also want to copy and paste the address URL which should be similar to the following:
http://localhost:6844/CustomerService.svc/
Downloading the tools…
In order to retrieve the data from an OData Data Source into Windows Phone 7, we are going to need to download the OData WP7 Library from CodePlex.
So, go ahead and navigate to http://odata.codeplex.com/.
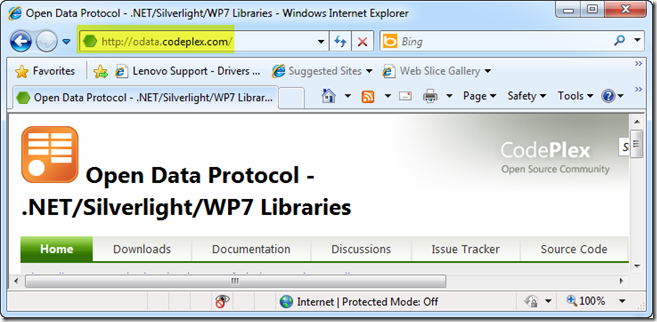
We are only interested in the binaries, so click on the Downloads page then look for Other Available Downloads and select ODataClient_BinariesAndCodeGenToolForWinPhone.zip as shown below.
After the download completes, you will have the following files:
An explanation is provided below:
- DavaSvcUtil.exe – is the utility that will create our class file from our OData Service.
- Readme.txt – provides a sample command line for creating the class file from the Netflix OData Data Service.
- System.Data.Services.Client.dll and System.Data.Services.Design.dll are the class libraries that we will add to our Windows Phone 7 Project.
Getting Started
Now that we have tested our OData Data Service and downloaded the tools, let’s get started by creating the proxy that our Windows Phone 7 Application will use.
We will need to go to a command prompt in order to generate our proxy. If you are using Windows 7 then you can quickly get to a command prompt by click Start –> and typing command in the search box.
Once you have a command prompt, simply navigate to the directory in which you extracted the directory to and type the following command:
datasvcutil /uri:http://localhost:6844/CustomerService.svc/ /out:.\CustomersModel.cs /Version:2.0 /DataServiceCollection
(Note: You will need the URI of the OData Data Service that we talked about in the first section of this article. I have bolded the paragraph at the top of this article that describes this.)
Quick Tip: You may also want to create a batch file and copy/paste the line above into it to quickly generate proxies in the future.
After this is complete, your screen should look similar to the following:
Notice that we have 0 errors and 0 warnings. If you have an error then step through each part of this tutorial again to make sure you have not left anything out. If all else fails, you can download a completed solution by scrolling to the top of this article.
If we navigate back into the folder that we extracted the .zip into, we will find a new file called CustomersModel.cs. This is our proxy that we will add to our Visual Studio 2010 Project.
Note: You will also note that I created a .bat file called “createclassfile.bat” to quickly generate this proxy again the future.
At this point, we are ready to create our Windows Phone 7 Application. So open up a new instance of Visual Studio 2010 and select File –> New Project.
Select Silverlight for Windows Phone –> Windows Phone Panorama Application and give it the name, SLShowODataP3 and finally click OK.
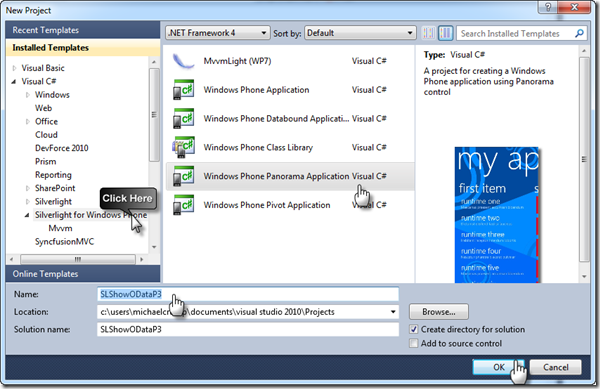
Once the project is loaded, the first thing we will need to do is add a reference to the System.Data.Services.Client.dll that we unpacked earlier.
Navigate to the directory containing the System.Data.Services.Client.dll file and double click it or select OK.
Now we will need to add the proxy that the DataSvcUtil.exe tool created.
Right click your project and select Add then Existing Item.
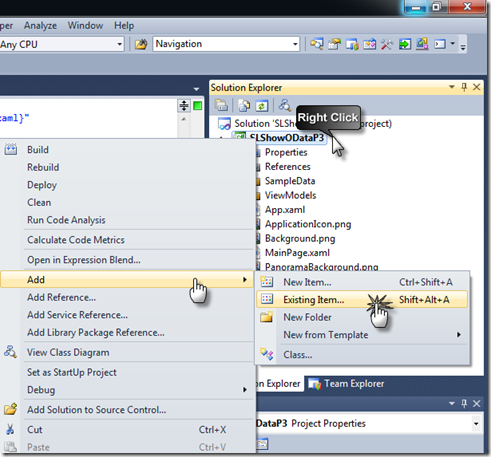
Navigate to the directory containing the CustomersModel.cs file and double click it or select Add.
Now we will need to create the User Interface and write the code behind to pull the data into our application.
Double click on the MainPage.xaml file and replace the contents of <—Panorama item one---> with the following code snippet:
1: <!--Panorama item one-->
2: <controls:PanoramaItem Header="first item">
3: <!--Double line list with text wrapping-->
4: <ListBox x:Name="lst" Margin="0,0,-12,0" ItemsSource="{Binding}">
5: <ListBox.ItemTemplate>
6: <DataTemplate>
7: <StackPanel Margin="0,0,0,17" Width="432">
8: <TextBlock Text="{Binding FirstName}" TextWrapping="Wrap" Margin="12,-6,12,0" Style="{StaticResource PhoneTextExtraLargeStyle}"/>
9: <TextBlock Text="{Binding LastName}" TextWrapping="Wrap" Margin="12,-6,12,0" Style="{StaticResource PhoneTextExtraLargeStyle}"/>
10: <TextBlock Text="{Binding Address}" TextWrapping="Wrap" Margin="12,-6,12,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
11: <TextBlock Text="{Binding City}" TextWrapping="Wrap" Margin="12,-6,12,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
12: <TextBlock Text="{Binding State}" TextWrapping="Wrap" Margin="12,-6,12,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
13: <TextBlock Text="{Binding Zip}" TextWrapping="Wrap" Margin="12,-6,12,0" Style="{StaticResource PhoneTextSubtleStyle}"/>
14: </StackPanel>
15: </DataTemplate>
16: </ListBox.ItemTemplate>
17: </ListBox>
18: </controls:PanoramaItem>
This xaml should be very straightforward. This ListBox contains a DataTemplate with a StackPanel that each TextBlock is binding to an element from our collection. This includes the FirstName, LastName, Address, City, State and Zip Code.
Now that we are finished with our UI and adding our Service Reference, let’s add some code behind to call our OData Data Service and retrieve the data into our Windows Phone 7 Application.
Now would be a good time to go ahead and Build our project. Select Build from the Menu and Build Solution. Now we can Double click on the MainPage.xaml.cs file and add the following code snippet, after replacing the MainPage() Constructor.
1: public MainPage()
2: {
3: InitializeComponent();
4: this.Loaded += new RoutedEventHandler(MainPage_Loaded);
5: }
6:
7: private void MainPage_Loaded(object sender, RoutedEventArgs e)
8: {
9: var ctx = new CustomersEntities(new Uri("http://localhost:6844/CustomerService.svc/"));
10: var coll = new DataServiceCollection<CustomerInfo>(ctx);
11: lst.ItemsSource = coll;
12:
13: coll.LoadCompleted += new EventHandler<LoadCompletedEventArgs>(coll_LoadCompleted);
14:
15: var qry = "/CustomerInfoes";
16: coll.LoadAsync(new Uri(qry, UriKind.Relative));
17: }
18:
19: void coll_LoadCompleted(object sender, LoadCompletedEventArgs e)
20: {
21: if (e.Error != null)
22: {
23: MessageBox.Show(e.Error.Message);
24: }
25: }
Lines 1-5 is the standard code generated except for the Loaded event. The Loaded Event created a new Event Handler called Main_Page Loaded.
Line 9 creates a new context with our CustomersEntities (which was generated by using the DataSvcUtil tool that we downloaded earlier) and passes the URI of our data service, which is located in our ASP.NET Project that we have running called CustomerService.svc.
Line 10 creates a DataServiceCollection which provides notification if an item is added, deleted or the list is refreshed. It is expecting a collection that was also created using the DataSvcUtil tool.
Line 11 sets the ItemSource of our ListBox to the collection.
Lines 15 simply creates a query that returns all records in our collection. We will modify this shortly to create a where and an orderby example.
Finally lines 19-25 are checking for an error and displaying a MessageBox. Of course, in a production application you may want to log that error instead of interrupting the user with a message they shouldn’t be concerned with.
If we go ahead and run this application, we would get the following screen.
Let’s add a query to filter the data. So go back to your MainPage.xaml.cs file and change this line:
1: var qry = "/CustomerInfoes";
to
1: var qry = "/CustomerInfoes?$filter=ID gt 3&$orderby=FirstName";
Now if we run this project you will notice that we only have 2 results returned and ordered by the FirstName. So in this example, Craig is listed first.
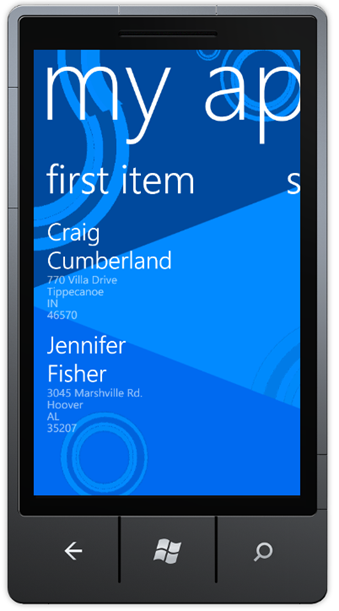
If you stop and notice that we are not using LINQ to produce the query where we were earlier in the Silverlight 4 Application. Instead we are writing the query manually. That is because this early implementation of OData on the Windows Phone 7 does not support the LINQ method in the current release. But do not worry as this will probably be addressed in a later release.
Conclusion
At this point, we have seen how you would produce and consume an OData Data Source using the web browser, LinqPad, Silverlight 4 and Windows Phone 7. We have learned how to do some basic sorting and filtering using all the same. Now that you are equipped with a solid understanding of producing and consuming OData Data Services, you can begin to use this in your own applications. I want to thank you for reading this series and if you ever have any questions feel free to contact me on the various souces listed below. I also wanted to thank SilverlightShow.Net for giving me the opportunity to share this information with everyone.
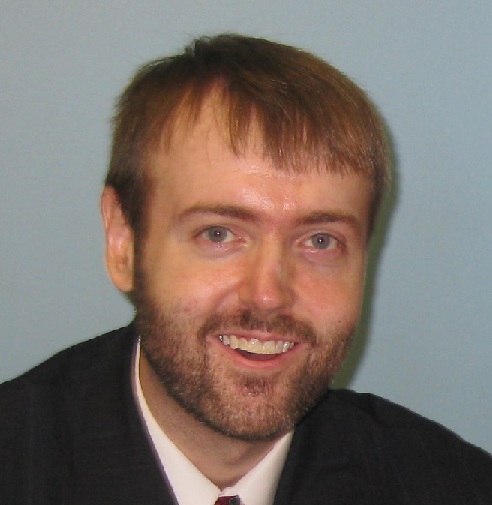
Michael Crump is an MCPD that has been involved with computers in one way or another for as long as he can remember, but started professionally in 2002. After spending years working as a systems administrator/tech support analyst, Michael branched out and started developing internal utilities that automated repetitive tasks and freed up full-time employees. From there, he was offered a job working at McKesson corporation and has been working with some form of .NET and VB/C# since 2003.
He shares his findings in his personal blog: http://michaelcrump.net and he also tweets at:@mbcrump