Welcome to a 3 part series on learning to develop Windows 8 applications using the MVVM pattern. MVVM stands for Model-View-ViewModel. The goal of this series is showing you how to get started with building a complete Windows 8 Store application based on this pattern.
In this first part, we will look at the pattern itself, describe the key concepts and look at a basic architecture setup. In the next part, we’ll start with the implementation of the basic concepts. In part 3, we’ll finish the series by adding the more advanced concepts. The final goal is having a base architecture that you can use as source of inspiration for your own Windows 8 applications. Let’s get started!
The code for the entire application can be downloaded at the top (note that this is the final application).
Data binding: where it all started
The MVVM pattern is based on the concept of data binding in Windows 8. Without data binding, it would be very hard to implement. Data binding is the concept that allows us to “bind” properties of controls to properties of data objects. Those objects are in-memory data: data binding has nothing to do with data access. It works with the result of a data access action (such as accessing a service).
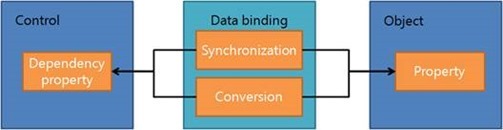
I’m not going to give you a complete overview of data binding; that would lead us too far. There’s a lot of information available on this topic. However, I do think a small list of things that are supported in Windows 8 Store app development data binding is in place:
- Base concepts of data binding, the syntax is the same
- Element binding
- Converters
- Model binding (DataContext)
- Indexers
- Data templating
- Binding to a CollectionViewSource
- Notifications using the INotifyPropertyChanged and INotifyCollectionChanged interface
- Single objects
- Collections
- Exceptions in output window
- Binding to anonymous objects
If you’ve been doing Silverlight 5 development, this means that a lot of features you may have been used already are not in there. This includes implicit data templates, custom markup extensions, breakpoints in XAML and quite a few more. While this is not good news, it’s certainly no disaster. The data binding options we have available in Windows 8 are robust enough to support MVVM!
MVVM: the pattern
Let me being by saying that MVVM is not tied to Windows 8. It works because of the data binding features that are brought to us by XAML. Now, other features such as commanding allow MVVM to work (we’ll introduce commanding later). As a pattern, it was introduced by John Gossman from the WPF. The pattern itself is based on MVC (let’s say it’s a variation of it) and ultimately, it’s based on the PresentationModel pattern. So it’s certainly nothing new!
The acronym MVVM stands for Model-View-ViewModel. Time to take a look at each of these 3 components and their interaction.
- Model: the model represents the data that we are working with. This can be a class that accesses a service and returns us the result (for example a service proxy). But it can also be a POCO (plain old CLR object), a class that builds up a list of data or basically any class that returns data in some way that we can use in the UI.
- View: the view is the visible part of the bunch. It’s the XAML code that we are using to show the UI to the user. We’ll be using data binding and commanding statements in the XAML code.
- ViewModel: the ViewModel is basically an abstraction of the View. Let me elaborate on that a bit more.
When we build an application without using the MVVM pattern, we end up with a lot of code in the code-behind. This includes event handlers (of course!) and quite a lot of code that interacts with the UI. This code also directly interacts with the model. The net result of coding this way is that it’s very hard to test the code in isolation. The code is dependent on UI elements and the interaction with these elements. We can say that this type of code is bad when it comes to SOC (separation of concerns): it basically does everything! Since we end up with a monolithic block of code, it’s hard to test parts of it too.
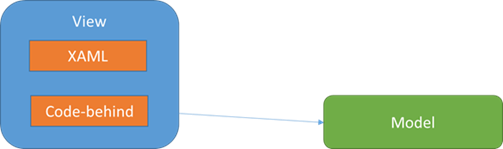
When we move to the MVVM, we are adding an extra layer of abstraction and that layer is the ViewModel. Take a look at the image below. We still have our view code (the XAML) but the code in the code-behind is reduced quite a lot (it’s not entirely empty though, some things should still be done in the view such as setting the focus; this is typical view code). The ViewModel layer now sits between the View and the Model. It will ask the Model to load in data and it will prepare this data so that the View can bind on it. To allow this, the ViewModel exposes state (in the form of properties), on which the View can then bind.
The ViewModel also contains the code that we originally would have placed in the event handlers. This code is now placed in Commands. Through the concept of Commanding, we can use data binding to link events happening in the UI with code in the ViewModel. We’ll see later on how this works.
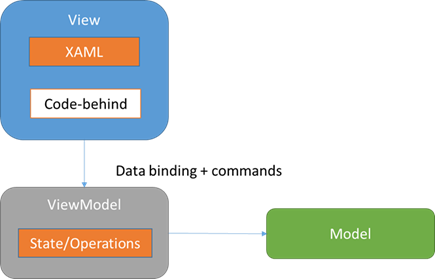
If we build up our code this way, we end up with a couple of independent blocks that can more easily be tested in separation. This also follows the SOC principle much more: each of these building blocks does one single things and is not trying to do everything!
It’s important to see who knows who in this setup. The View of course knows the ViewModel: it needs to bind on it. The ViewModel however doesn’t know the View. Similarly, the ViewModel knows the Model (it needs to ask it to load data for example) but the Model doesn’t know the ViewModel. This chain again confirms what we’ve seen earlier: we are building components that are not hard-linked to each other. This way, we can easily introduce a mock to test any of the components in isolation.
So in short, the ViewModel connects the View and the Model. It contains state (properties) that is directly usable by the View through data binding. There is however no hard link between the View and the ViewModel (this would again break the testability). It is as said before, an abstraction of the View. In any case, your ViewModel can never have references to controls that live in the View. If you see that you’re ending up with this, you’ve done something wrong at some point!
Should you not have code in your View’s code-behind at all then? Some say no. I beg to differ. Code that is not doing things with the model but is directly linked to the View, should be in the View. For example, focus setting should be done in the View: this has nothing to do with the model.
My framework of choice: MVVM Light
Now how do you get started with building a Windows 8 Store app based on MVVM? Well, you can start from scratch. However, there are some frameworks already out there that get you on your way faster. I’m not going to spend time on showing you all these frameworks (these articles teach you about developing in MVVM, not the frameworks themselves), so I’m going to use my personal favourite for this demo: MVVM Light (available via http://mvvmlight.codeplex.com). The reason I like this framework is that it’s very lightweight (hence the name). It simply contains a couple of base classes (such as a base ViewModel, a ViewModelLocator and quite a few more) that you can use to jump-start your MVVM development.
The most important building blocks of MVVM Light are:
- ViewModel
- Commands
- RelayCommand
- EventToCommand
- Messaging
- Messenger
- Several types of messages
- IOC
It’s also easy to take out one of these and replace it with your own implementation (for example if you want to use another IOC container, you can do so without any problem).
The sample application
By now, you should have a basic understanding of the concepts behind MVVM. It’s time to look at a sample implementation. The application we’re using for this demo is a simple ToDo application. The user can get a list of ToDo items, as shown below.
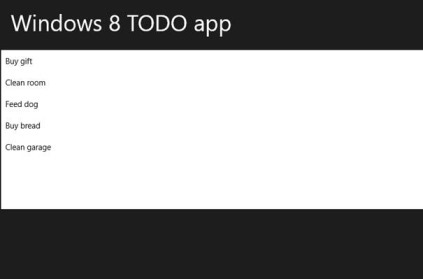
When clicking on an item, we can see the detail of the item.
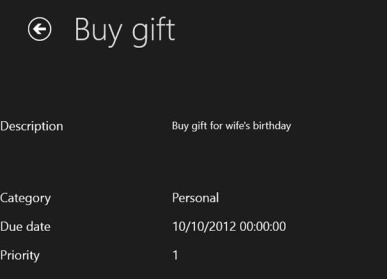
From this detail page, we can delete the item as well.
Architecture of the application
The solution contains a number of projects. Below I’ve pasted a screenshot of the Solution Explorer.
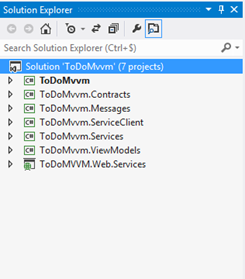
We’ll dive in the code later, for now it’s important to see what the different projects contain and what their function is.
- ToDoMvvm: contains the Windows 8 Store app itself. The project is built using the MVVM pattern. Of course, we’ll look at the code of this one in much more detail!
- ToDoMvvm.Contracts: contains several interfaces. The application is built using IOC, we’ll use the contracts contained in this project to make this possible. This project is referenced by both the ToDoMvvm and ToDoMvvm.ViewModels. This way, these 2 projects can work with interfaces declared in this Contracts project without creating hard links to concrete types. This ensures that all components are loosely coupled and increases the ability to test the different items in isolation.
- ToDoMvvm.Messages: contains the message classes to make message-based communication between ViewModels possible. We’ll see later that messages are the preferred way of communication between the ViewModels.
- ToDoMvvm.ServiceClient: contains the service proxies for the generated service references. Simply adding a service reference to ToDoMvvm.Web.Services is enough here. I place this in a separate project although that’s not required of course. I did this to make things as clean as possible.
- ToDoMvvm.Services: contains the service classes such as a Data Access Service. The different services that I’ve included here will be discussed later on. For now, just think of these service classes as simple classes that perform just one task (think SOC!)
- ToDoMvvm.ViewModels: contains the ViewModel classes.
- ToDoMvvm.Web.Services: the application retrieves the list of ToDo items from a WCF service. This service is contained in this project.
Summary
In this first part, we’ve explained the concepts of MVVM and shown the solution structure. In the next part, we’ll start diving in the code and look at the different implementation choices!
About the author
Gill Cleeren is Microsoft Regional Director (www.theregion.com), Silverlight MVP (former ASP.NET MVP) and Telerik MVP. He lives in Belgium where he works as .NET architect at Ordina (http://www.ordina.be/). Passionate about .NET, he’s always playing with the newest bits. In his role as Regional Director, Gill has given many sessions, webcasts and trainings on new as well as existing technologies, such as Silverlight, ASP.NET and WPF at conferences including TechEd Berlin 2010, TechDays Belgium – Switzerland - Sweden, DevDays NL, NDC Oslo Norway, SQL Server Saturday Switserland, Spring Conference UK, Silverlight Roadshow in Sweden, Telerik RoadShow UK… He’s also the author of many articles in various developer magazines and for SilverlightShow.net and he organizes the yearly Community Day event in Belgium. He also leads Visug (www.visug.be), the largest .NET user group in Belgium. Gill is the author of “Silverlight 4 Data and Services Cookbook”. In 2012, the second edition, “Silverlight 5 Data and Services Cookbook” was released.
You can find his blog at www.snowball.be.
Twitter: @gillcleeren