In the previous article, we’ve introduced contracts in Windows 8. To quickly recapitulate, a contract allows Metro style applications in Windows 8 to communicate which each other or with Windows, without there being a hard reference between the participants of the communication. We’ve seen how the search contract allows applications to open themselves up to Windows so they can be search. There’s of course a contract that specifies how this searching is to be done: an application has to follow the rules specified in the contract so that Windows can communicate with the app in the way it needs to do.
In this article, we’ll dive some more in contracts, more specifically, the share contract.
The code for this article can be downloaded here.
We love sharing
Sharing information has become something very trivial. Millions of sites out there have sharing options. “Share this on Twitter”, “Post on Facebook”, “Share via mail” and tons of other options have been popping up everywhere on the internet. Why? People love sharing. When they find something cool or they’ve just read the most interesting article, people want to share that with their contacts. Perhaps they want to broadcast it, using a social network. Or perhaps they want to target a specific contact and send the information via mail. Also, people sometimes want to share information related to something they have done or found inside an application. Perhaps they’ve achieved a high-score in some game and they want to brag with it. Or, they have found a great recipe in a cookbook application.
As you can see, sharing is all around us. One problem though is the fact that it’s sometimes still too hard for people to quickly share the information they want to share. As said, a lot of sites have embedded sharing icons. But are they always easy to find? Or what happens if you use a social network that the site you’re using isn’t listed? In that case you need to go and paste the link to the social networking site. Or as mentioned with the game high-scores: if the game doesn’t support sharing, how are you going to proof that you’ve really attained that high-score? Perhaps through a screenshot that you manually have to take, copy and paste to another site or application.
From a developer’s perspective as well, implementing the right sharing options can be hard. Sites offer often quite a few sharing options and maintaining all these external links can be a time-consuming task. What if tomorrow Twitter decides they want to implement that option differently? You as the developer need to go and change your implementation. And even then, perhaps the visitor of your site (or user from your application) uses some obscure social network (perhaps one that’s relevant in his home country) that you haven’t implemented…
Microsoft thought about offering an integrated sharing experience in Windows 8. It’s built into the system to offer both the end-user and the developer an easy and universal way of sharing information. This is the share contract.
The share contract
Through the share contract, Windows 8 makes it possible for 2 applications to share information with each other. The big plus for developers is that the 2 application don’t have to know each other. Sharing is always done between two applications: the share source and the share target. The share source will share information in a specific format defined by the contract; the share target will be getting that information through Windows. Since the information is in a specific format, the developer of the share target can anticipate on this and write his code based on this format.
Note that Windows is the mediator between the two apps. It handles the process of getting the information from the share source and getting it to the share target. It’s safe to say that we have some kind of pub/sub architecture.
Let’s take a look at the share contract in action. Below you can see the CookBook application. I’m not very much of a cook but I can image that people would love to share a recipe with someone via mail or perhaps on Facebook. From within the Cookbook application, we use the Charms (swipe in from the right or use Win + C) and select Share.
In most apps (at least from the list of apps currently available), it’s the developer who has chosen what we can share. In the CookBook app, it’s created this way as well. Of course, the developer of the application can also select to allow the user to specify the information he wants to share. After clicking/tapping on Share, the list of share targets appears. If you’ve read the previous article, it’s easy to see a similarity between this and the list of apps that registered for Search. Indeed, being a Share target is done through a declaration (as we’ll see later in this article). Based on the contents of the data being shared, this list is filtered automatically by Windows.
Now that the user made a selection, the share target app opens. In most cases, the app doesn’t open in its default view. Instead, it opens a specific view for handling the sharing operation. Below, we see the SDK Sample Target application accepting the share data.
Let’s now look at how we can create both an application that is a share source and an application that is a share target. We’ll start with the source.
Making your application a share source
Implementing the share contract as source in your application comes down to the following steps:
- Via code, registering your application with the DataTransferManager.
- Your application should register itself for the DataRequested event of the DataTransferManager.
- Filling up the DataPackage when the above mentioned event is fired by Windows
Let’s look at these steps in a sample application. Create a new C# Metro style application and name it MyShareSource. The UI is nothing more than a basic Button that triggers the display of the Share UI. We are going to share basic text here. The UI of the app is shown below.
The registration with the DataTransferManager is done in a separate method named RegisterForSharing() which we’ve called from the constructor. We retrieve the DataTransferManager and then register for the DataRequested event. This event will be triggered when the user indicates he/she wants to share data from the application. This event is triggered by Windows; in fact, it is triggered by the Share Broker. The Share Broker is the component that sits between the source and the target applications. The first thing it does is handling the incoming sharing request (triggered by the user willing to share) and routing it to the source application. Below we see the RegisterForSharing() method.
public BlankPage()
{
this.InitializeComponent();
RegisterForSharing();
}
private void RegisterForSharing()
{
DataTransferManager datatransferManager;
datatransferManager = DataTransferManager.GetForCurrentView();
datatransferManager.DataRequested += newTypedEventHandler<DataTransferManager,DataRequestedEventArgs>(this.DataRequested);
}
In the DataRequested event, we create the something called the Data Package. This can be seen as a container for data; it contains the data that the source application shares with the target. It can contain several formats, varying from simple text and URIs to images and even custom data formats. The latter should mostly be used if you want to target a specific share target app (for example, you’ve created 2 Metro style apps between which you want to exchange data). You can add more than one type of format (for example, an image, text, URI and a custom format all at once). Based on the contents, the Share Broker will filter the list of target applications. Below is the code for the DataRequested event where we build up the Data Package.
void DataRequested(DataTransferManager sender, DataRequestedEventArgs e)
{
e.Request.Data.Properties.Title = "Sample sharing source";
e.Request.Data.Properties.Description = "This is some Lorem ipsum text";
e.Request.Data.SetText(ShareContent.Text);
}
Run the application now and notice that when clicking, you’ll see that the Share UI is shown. If you have any apps registered as target, you can go ahead and share the text.
Now that we can share data, let’s see what we need to do to accept data.
Making your application a share target
We’ve seen that the Share Broker has asked the source application to create the Data Package. Once ready, it will inspect it and look at the different data types included. Based on this, the long list of all registered target apps is filtered to only those that can handle at least one of the included types. This is something we don’t have to worry about, it’s done for us.
When the user selects an application, the Share Broker will start the target app. It gets activated (remember the search activation from the last article) and gets passed in the data package (the target app gets read-access). The target app can (but is not forced) to send a completion so that the source app knows it can continue its normal run.
Notice that the sharing experience is in-context, meaning that the user doesn’t leave the application to go and copy/paste some information in another application!
Let’s see how we can make this working. Create a new Metro style app and name it MyShareTarget.
First, add a new Share Target Contract from the Project template window and name it SimpleShareTarget.
Adding this template does a number of things. First, it adds a declaration to your manifest: the Share Target declaration. Based on this, Windows will know that your application can be the target of a share operation. We need to specify the types of content, shared via the Data Package, we want to receive. Since we are sharing text in the MyShareSource application, we specify that as the only type here.
Secondly, your App.xaml.cs is modified: it overrides the OnShareTargetActivated, accepting a ShareTargetActivatedEventArgs instance. The code is shown below.
protected override void OnShareTargetActivated(Windows.ApplicationModel.Activation.ShareTargetActivatedEventArgs args)
{
var shareTargetPage = new MyShareTarget.SimpleShareTarget();
shareTargetPage.Activate(args);
}
Notice what this code does: it doesn’t load the default start page, instead it activates a new page of type SimpleShareTarget. The code for this Activate() method is also generated. We need to make some changes to it so that the shared text will also appear. Below is the code for this method.
public async void Activate(ShareTargetActivatedEventArgs args)
{
this._shareOperation = args.ShareOperation;
// Communicate metadata about the shared content through the view model
var shareProperties = this._shareOperation.Data.Properties;
var thumbnailImage = new BitmapImage();
this.DefaultViewModel["Title"] = shareProperties.Title;
this.DefaultViewModel["Description"] = shareProperties.Description;
this.DefaultViewModel["Image"] = thumbnailImage;
this.DefaultViewModel["Sharing"] = false;
this.DefaultViewModel["ShowImage"] = false;
if (_shareOperation.Data.Contains(StandardDataFormats.Text))
{
this.DefaultViewModel["MainText"] = await _shareOperation.Data.GetTextAsync();
}
Window.Current.Content = this;
Window.Current.Activate();
// Update the shared content's thumbnail image in the background
if (shareProperties.Thumbnail != null)
{
var stream = await shareProperties.Thumbnail.OpenReadAsync();
thumbnailImage.SetSource(stream);
this.DefaultViewModel["ShowImage"] = true;
}
}
We also need to update the UI for the SimpleShareTarget.xaml view so that we see the text.
<Grid Grid.Row="1" Grid.ColumnSpan="2">
<TextBlock
Text="{Binding MainText}"
Foreground="{StaticResource ApplicationSecondaryTextBrush}"
Style="{StaticResource BodyTextStyle}"/>
</Grid>
Now run the MyShareTarget app. You won’t see anything, since now the BlankPage.xaml is running. Behind the scenes though, your app has registered itself to be a share target.
To see that in action, run the MyShareSource application again. Now when you click on Share, you’ll see your application listed. Select it and watch your content being shared with the target application!
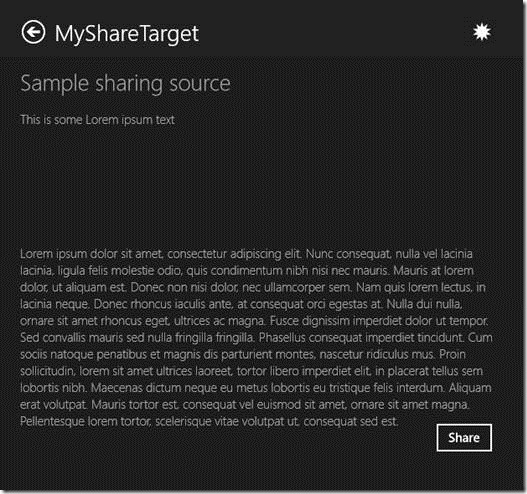
Summary
In this article, we’ve taken a look at a second interesting contract in Windows 8: the sharing contract. It allows two applications to communicate with each other without there being a hard reference between the two.
In the next article, we’ll dive into the creation of tiles! Stay tuned!
About the author
Gill Cleeren is Microsoft Regional Director (www.theregion.com), Silverlight MVP (former ASP.NET MVP) and Telerik MVP. He lives in Belgium where he works as .NET architect at Ordina (http://www.ordina.be). Passionate about .NET, he’s always playing with the newest bits. In his role as Regional Director, Gill has given many sessions, webcasts and trainings on new as well as existing technologies, such as Silverlight, ASP.NET and WPF at conferences including TechEd Berlin 2010, TechDays Belgium – Switzerland - Sweden, DevDays NL, NDC Oslo Norway, SQL Server Saturday Switserland, Spring Conference UK, Silverlight Roadshow in Sweden, Telerik RoadShow UK… He’s also the author of many articles in various developer magazines and for SilverlightShow.net and he organizes the yearly Community Day event in Belgium. He also leads Visug (www.visug.be), the largest .NET user group in Belgium. Gill recently published his first book: “Silverlight 4 Data and Services Cookbook” (Packt Publishing). His second book, Silverlight 5 Data and Services Cookbook will be released early 2012. You can find his blog at www.snowball.be.
Twitter: @gillcleeren