Are you interested in
creating games for Windows Phone 7 but don't have XNA experience?
Watch the recording of the recent intro webinar delivered by Peter Kuhn '
XNA for Windows Phone 7'.
This article is Part 0 of the series “XNA for Silverlight developers”.
This article is compatible with Windows Phone "Mango".
Latest update: Aug 1, 2011.
Introduction
Around the time the first Windows Phone 7 devices were released to the market, one popular sentence you heard was "every Silverlight developer is a Windows Phone 7 developer" – and that's true. Silverlight is Microsoft's main platform to do Windows Phone 7 development, and every desktop Silverlight programmer will feel comfortable in the new mobile programming environment instantly. Sure there are differences and libraries specific to the devices, but you won't have to learn a new programming language or new ways to define your UI, and you can use the same development environment you've been using for normal Silverlight development all the time. That's great!
However, Silverlight is not the only possibility to create software for Windows Phone 7 devices, there's also XNA. Most people that are interested in developing for the new mobile platform probably have already read about it, mostly in the context of game programming. This article tries to give a quick overview of XNA on the mobile platform for Silverlight developers who have not dealt with XNA in detail before. It explains the possibilities of interaction between Silverlight and XNA, and why you as a Silverlight developer should care about XNA even if you don't want to develop games.
In successive parts of this series, we'll go into the details of programming 2D games for Windows Phone 7 using XNA, with special focus on those parts that require a change of thinking when you are coming from a Silverlight background. I strongly encourage you to comment on each part and request more information about topics you are interested in. If possible, your feedback will directly result in the design of future articles.
The Windows Phone 7 Platform
The base of all development on the Windows Phone 7 platform is a .NET Framework managed runtime code sandbox based on the Compact Framework know from previous mobile platforms (Windows CE/Mobile). This means that all application development for the phone devices is done in managed code and in a protected sandbox. On top of that, the following structure is established:
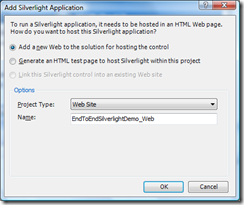
As you can see, both Silverlight and XNA share a great amount of the available functionality. The base class library provides all the basic plumbing needed in most software and is similar to what you know from other platforms like desktop Silverlight. Neither this base class library nor most of the provided services specific to the Windows Phone 7 platform are tied to Silverlight or XNA per se. In fact, it's even possible for great parts of Silverlight and XNA to interact with each other and to be used side-by-side. Let's see how that works.
XNA and Silverlight Interop
When a .NET or Silverlight developer reads "interop", they often think of painstaking work ahead. For Win32 interop, you need to import operating system functions, map data types and marshal between the managed and unmanaged world. For COM interop in Silverlight, you need to know the API by heart or look up every detail in its documentation, as you don't have any built-in help like Intellisense in Visual Studio which could guide you through the process of interacting with the unmanaged component. In this situation however, things are different.
Since all development for Windows Phone 7 happens to be managed code in C# or Visual Basic .NET, and both Silverlight and XNA manifest themselves as libraries which can be referenced and accessed natively in managed code, the borders between both are easily blurred. The transition between both worlds in fact is so seamless that developers often don't even realize when they cross these borders. It's likely that even if you as a Silverlight developer have never consciously used XNA, an application of yours did, behind the scenes, without you even noticing.
A concealed example
A very prominent example for this is the Gesture Service and Gesture Listener that can be found in the Silverlight for Windows Phone Toolkit. These classes provide an easy way to access gestures like flicks or pinching in your Silverlight application. When you look at the source code however, you will realize that this construct is only a shallow wrapper around the Input.Touch namespace functionality from the XNA framework. It adds some logic to recognize gestures and to translate from XNA's polling-based model to the event-driven nature of Silverlight. To many people this comes as a surprise, and they're not aware it's actually XNA when they're using these features.
Limitations of Silverlight
In some areas we also have some limitations in Silverlight when it comes to making full use of the phone device features. In these cases, it's a good idea to take a quick look at XNA, because sometimes it offers superior possibilities in the same areas, which simply are not available in Silverlight. A very simple example of this is the message box. Silverlight's API for this is very limited:
MessageBox.Show("Hallo World",
"This is a caption",
MessageBoxButton.OK);
This is about all you get. You can show a text with a caption, and decide whether you want to show the OK button or both OK and Cancel buttons. In XNA though, there is the Guide helper class, which let's you do something like that:
Guide.BeginShowMessageBox("This is a caption",
"Hallo World",
new string[] { "First button", "Second button" },
1,
MessageBoxIcon.Alert,
null,
null);
You can specify the number of and labels for the buttons yourself, and when you pass in different values for the MessageBoxIcon argument, you will get different behaviors for your message box (e.g. different sounds). Another thing to note is that this message box is non-blocking by default, which is also not possible in Silverlight; but it also means you have to put extra effort into your code if you want to make it blocking.
Other areas where XNA offers features that are not available in Silverlight are:
- Certain audio features like playing multi-channel sounds.
- Advanced sound effects, for example changing parameters like pitch and similar on the fly while the sound is playing.
- Recording audio using the phone's microphone.
- Interaction with the media library to load songs, videos and pictures (without using the PhotoChooserTask) and also to save pictures back to the media library.
- The previously mentioned input gestures, which are now also available by using the Toolkit wrapper.
Limitations of interop
Although you can use great parts of both worlds in the same application, there are some restrictions to the possibilities of interop between XNA and Silverlight. To be more specific, the official certification requirements for the RTM release published by Microsoft contain a paragraph that says:
4.2.5 The application must not call any APIs in the Microsoft.Xna.Framework.Game
assembly or the Microsoft.Xna.Framework.Graphics assembly when using any methods from
the System.Windows.Controls namespace.
That's a heavy one. A lot of developers would love to pick the best of two worlds and mix visual elements from XNA and Silverlight in their applications. XNA does not have a real concept of controls, and hence does not ship with a set of built-in UI controls, so it would be great to be able to use Silverlight controls for your game's menu system, for example. And the other way round, a great benefit for Silverlight developers would be to use XNA in parts of their application, for example when they need the superior rendering capabilities and performance, or native 3D support. Unfortunately, with the above restriction in place, none of this is possible in the RTM release.
The good news however is that this limitation is eased in the first major update of the platform (code name "Mango"). Starting with this version of Windows Phone, developers will be able to mix XNA and Silverlight in the same application. There still are restrictions in place (for example, you still won't be able to use anything from the Game assembly), and doing this requires to choose a certain solution/project setup where Silverlight takes the leading role; however, it allows you to use the best from Silverlight and still have XNA rendering parts of your game or application, or even mix elements of both technologies on the same page. We will see how this works in more detail in part 12 of this series.
Windows Phone 7 as a Gaming Platform
From the beginning, Windows Phone 7 has aggressively been promoted as a gaming platform. Big players in the industry like Electronic Arts are developing games for this platform, and you will find a lot of well-known brands in the market place, from The Sims™ to Assassin's Creed™ to Guitar Hero 5 and a lot more. In 2011's CES keynote from Steve Ballmer, a big part of the Windows Phone 7 presentation was the show case of current and future games. Obviously gaming is a major part of Microsoft's strategy for these devices.
For smaller companies or hobby developers, the mobile platform offers the opportunity to develop casual games people can play wherever they are. Some very remarkable games are already available and prove that an addictive idea does not necessarily need a big publisher or developer team to make a successful appearance on the phone. Game development apparently is an area developers for Windows Phone 7 are very interested in. At the moment of writing this, the market place offers around 5900 apps for download, and out of this 1340 are games. This makes games by far the category with the most entries:
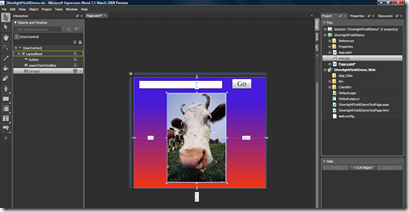
In the following parts, I'll go into the details of what considerations you need to make when you want to develop games for Windows Phone 7.
Choosing between Silverlight and XNA
Because XNA is a dedicated framework for game development, a lot of people perceive this as the only possibility to create games for Windows Phone 7; some even think it is a certification requirement to use XNA when you want to publish applications to the game section of the market place. This could not be more far from the truth. You are free to also choose Silverlight as your development tool for game creation. In fact, I know of several games in the market place that are made with Silverlight, and your decision on what technology to use should be made carefully to optimize your development process in terms of efficiency, depending on your requirements.
When to choose XNA
3D support – This is a major argument for XNA. If you need that extra dimension, it's almost certain that XNA which is built on Direct3D will be the better choice. You have excellent support for 3D graphics with it, and if you have worked with 3D technology before (not necessarily XNA, but also native Direct3D or even OpenGL) the involved concepts and theory will be very familiar to you and make the transition easy. Although it has some limitations (for example in terms of shader support), XNA on the phone basically has the same features as Direct3D 9. Silverlight on the other hand has no real support for 3D, and it would require a lot of work to even get some of the basic things done.
Performance – When you are about to create a visually complex game, probably with a lot of effects and moving elements, it's advisable to use XNA. It has been optimized for performance and GPU rendering. Silverlight's layouting system is more comfortable, but that comes at the cost of lower performance in these situations, and when you have to do a lot of blending and work with transparency a lot (particle effects and the like), performance may suffer with Silverlight.
Compatibility with XBox – If you are planning on developing a game for both the Phone and XBox or even the Windows Desktop, and to share code between those implementations, Silverlight wouldn't be an optimal choice as it is not available on the XBox. XNA is not only a supported framework on the Phone, but also runs on the XBox and Windows desktop systems, which makes it considerably easier to share code for projects targeted at those platforms.
Misc – There are some more smaller arguments for XNA. Using the built-in content pipeline to import assets provides a more powerful mechanism to organize your game content, for example. It supports a variety of formats for graphics and sounds that can be imported and converted without additional work, and you can even write your own importers for custom formats that integrate seamlessly into your developer experience if you like.
When to choose Silverlight
Comfort – Silverlight's development experience, especially compared to XNA, is a pleasure in a lot of situations. The design-time support of tools like Expression Blend alone can be a huge boost in productivity. That story continues when it comes to the rich set of built-in controls you can use in Silverlight (buttons, text controls, items controls etc.); you have to create those all manually in XNA, from scratch, if you need something comparable. Then there's the layout system and controls that do all the heavy lifting for you when it comes to arranging content automatically – in XNA you'll find yourself calculating positions and coordinates for these things a lot. So if your game depends a lot on user interaction with standard controls, or you can make good use of the built-in Silverlight features or use the available tools to cut your development time in half, Silverlight should definitely be a consideration for you.
Compatibility with the web browser – We have talked about how XNA is great when you want to develop for both the phone and XBox. The same is true for Silverlight if you aim to create a game for both the phone and the web. It is unlikely that you can reuse your UI for a desktop Silverlight application. But again, if you plan on sharing code between the two platforms and plan ahead, Silverlight might be the better choice, as XNA's programming model is so different from Silverlight's and hence harder to port to a web application.
Vector graphics – Silverlight's rendering system is vector based, even the text. That means that you get some benefits from that for free, for example lossless scaling capabilities. XNA has little to no support for this (text rendering is also bitmap based). So if your game heavily depends on zooming of elements that can be designed as non-bitmaps in Silverlight, you might want to make use of this.
Misc – As with XNA, there are also minor arguments for Silverlight for specific scenarios. For example, if you want to work with video content, then Silverlight offers great flexibility with the media element. XNA on the other hand only supports full-screen video playback, which means you cannot embed video nicely into your application.
When to choose both
As mentioned above, starting with Windows Phone "Mango", you will be able to mix both technologies in the same application. This is an attractive alternative for a lot of scenarios, and it allows you to easily make use of the best from XNA (like 3D support, the better performance, the content pipeline and more) and Silverlight (the comfort of having a layout and animation engine, pre-built controls, vector graphics and other things) at the same time.
You may ask yourself why you shouldn't always use this kind of integrated application. One major argument against this is the worse portability of your code. XNA is a technology that supports not only Windows Phone, but has been established for years on other platforms like the PC and XBox. If you want to target those other platforms with your game too ("three screens"), then one of your goals of course is to reuse as much code as possible. Once you have decided to make use of the Silverlight-XNA-integration of Mango though, your code will need some adjustments (like not relying on the Game class anymore and others), and that will likely make it harder to achieve this goal.
Another point of course is that this feature requires your end users to have Windows Phone "Mango" on their devices. The rollout of this new version only starts in the near future, and it will surely take months from now for the majority of users to transition to it. Until then, if you target Mango features like this exclusively, you are limiting the reach of your application and the number of potential customers. Of course this is only a temporary issue that will be solved over time, but depending on your release schedule it might be a big issue.
Summary
In this article we have learned that Silverlight and XNA, although very different in their programming models, are not that far apart on Windows Phone 7. Both are based on a common foundation, and parts of the libraries can be and already are shared between those two worlds easily.
We have also seen that game development for the new mobile platform is not something exclusive to XNA, but can be accomplished using Silverlight too. In fact, both technologies have their advantages when it comes to effectively programming games for Windows Phone 7.
I hope that by giving a glimpse of XNA, I've sparked some interest for those who had no motivation to delve into it so far. In the following parts of the series, we will shed light on several aspects of programming games with XNA and also focus on the differences to Silverlight's programming model to ease the learning curve for those who didn't engage in XNA development yet.
Please feel free to add your comments and thoughts, or contact me directly with any questions you have.
About the Author
Peter Kuhn aka "Mister Goodcat" has been working in the IT industry for more than ten years. After being a project lead and technical director for several years, developing database and device controlling applications in the field of medical software and bioinformatics as well as for knowledge management solutions, he founded his own business in 2010. Starting in 2001 he jumped onto the .NET wagon very early, and has also used Silverlight for business application development since version 2. Today he uses Silverlight, .NET and ASP.NET as his preferred development platforms.
He created his first game at the age of 11 on the Commodore 64 of his father and has finished several hobby and also commercial game projects since then. His last commercial project was the development of Painkiller:Resurrection, a game published in late 2009, which he supervised and contributed to as technical director in his free time. You can find his tech blog here: http://www.pitorque.de/MisterGoodcat/