Introduction
The Microsoft’s Live SDK (or commonly referred to as Live Connect), provides a set of controls and APIs that enables applications to integrate single sign-on (SSO) functionality using Windows Live ID. You can also use it to access data in SkyDrive, Hotmail, and Windows Live Messenger.
The library supports multiple platforms, including Windows Phone 7 Mango and Windows 8 Metro Style applications using either C#, Visual Basic or JavaScript. In this article, we will build our first metro style application using the Live SDK and XAML/C#.
Download the Bits
Let’s begin by downloading the following items:
- You will need to download the Windows 8 Developer Preview to follow along with this article. This will install Windows 8 along with the new Visual Studio 11 which is required to build Metro applications.
- Download the Live SDK v5.0 or higher - The Live SDK provides a set of controls and APIs that enable applications to integrate single sign-on (SSO) using Windows Live ID and to access data in SkyDrive, Hotmail, and Windows Live Messenger.
- Optional, but highly recommended - The Metro Style App Samples which provides various sample metro applications for future reference. It is licensed for public use, so feel free to use it in your own applications.
- Optional, but recommended - Read the Windows 8 Developer Preview guide to start learning about the features/functionality coming in Windows 8.
Signing into Windows 8 Using your Windows Live ID
One of the most important things to learn before building your first application that uses the Live SDK is the various ways to sign into your Windows 8 machine.
You currently have two ways to sign into your Windows 8 machine and each way determines the prompts the users will receive when launching a application that uses the Live SDK.
- A Local Account – which stores all of the data regarding your profile on the local machine.
- If you user is using this method, then they will get the prompt to sign in and to allow access.
- A Windows Live ID – retrieves profile information directly from your Live ID and enables features such as roaming and easy sign-on to Microsoft services.
- If you user is using this method, then they will only get the prompt to allow access.
In this tutorial, we will be signing into Windows 8 using our Live ID.
If you have already setup a “Local Account” then you can switch very easily by going to Control Panel –> Users then clicking on “Switch to a Windows Live ID” as shown below.

You will then be prompted to enter your current password of the local account and log in using your Live ID username/password. If you don’t have one then you may sign up for one through this wizard.
Immediately following you should receive an email similar to the one below:
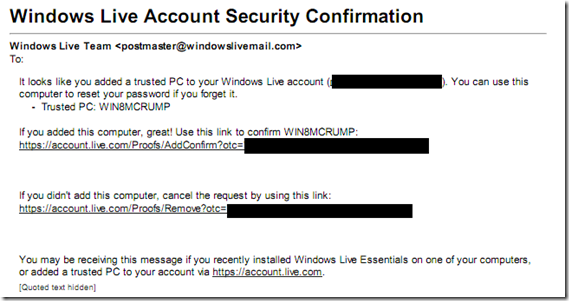
If you click on the link to confirm your identity then a web browser will pop open and you will be greeted with “Manage Security Info”. This screen will list mobile devices, email address and trusted PC linked to your account. As we can see below that I have three Windows 8 machines linked to my account.
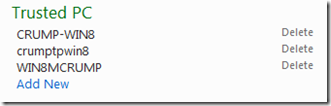
You can also add a new trusted PC manually with the “Add New” link. To use this feature, you must access your account using Internet Explorer and have Windows Live Essentials installed.
Let’s Get Started
Now that we have the necessary tools to begin building a Metro Application using C#/XAML and we we have signed into Windows 8 using our Live ID, let’s begin.
In this sample application, we are going to retrieve the following information from the users profile:
- Users First and Last Name
- What gender they are and what region they live in
- URL to their Live Profile
As well as add a contact to their address book.
Launch Visual Studio 11 Developer Preview and select Visual C# -> Windows Metro style –> Application. Give the project the name MyFirstLiveConnectApp and hit OK.
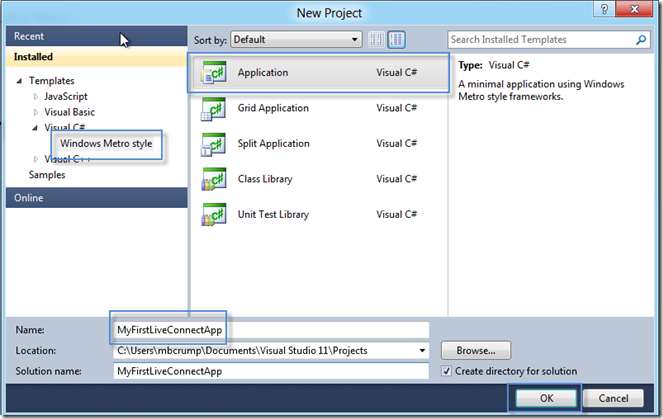
Adding the Live SDK References
In case you haven’t already, please download and install the Live SDK v5.0 before proceeding.
Once that is complete, we can add the references to our project by Right Clicking on References inside of Visual Studio 11 and selecting “Add Reference”. Next, you will need to select Windows –> Extension SDKs –> Live SDK then Add.
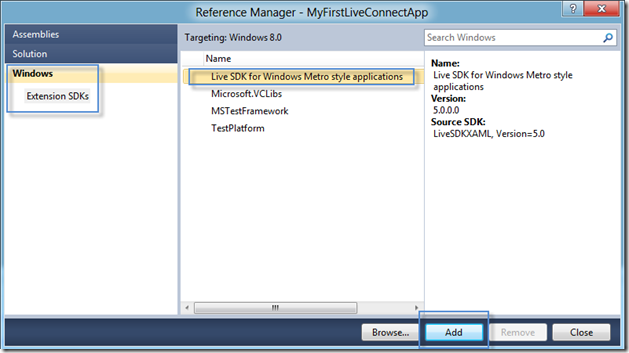
Registering the Application with Windows Live
Before we begin building this app, you need to register it with Windows Live. You can register your app by going to the application management site and following the instructions on the site.
The only item that you want to pay special attention to is the Package Name in Step 3 as you will copy and paste that into your Application’s Package.appxmanifest file. (A walk-through is included in that site).
If you don’t register your application and try to use Live services, then your user will be presented with the following error message.
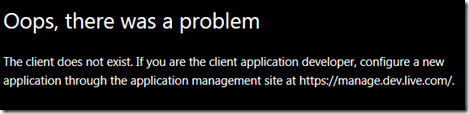
Once this process is complete you can view your registered applications here. This page will contain all the API settings needed to use the Live SDK in your projects.
Understanding what is under the hood…
When we select the C#/XAML Application template, Visual Studio 11 provides us with basic application structure. Before we begin building our own, it makes sense to spend a few minutes examining the XAML pages included in the existing structure.
- References – It is empty by default because Microsoft decided that all the WinRT assemblies are always referenced by default and they have no reason to display them. Any of your own referenced assemblies or third party references will be shown as in this case our Windows Live SDK is shown.
- App.xaml is used by Metro applications (amongst other XAML technologies) to declare shared resources like brushes, various style objects etc. The code behind file of App.xaml is used to handle global application level events like OnLaunched and OnActivated.
- MainPage.xaml contains the markup of what our user interface will look like once the application is executed. The default Metro MainPage.xaml file consists of a UserControl and a Grid.
- Package.appxmanifest – Is basically an XML file, but if you double click it then you will notice four tab panels inside it (named: Application UI, Capabilities, Declarations and Packaging)
Back to Building a Simple User Interface
Here is a mock-up screen of what our app will look like:
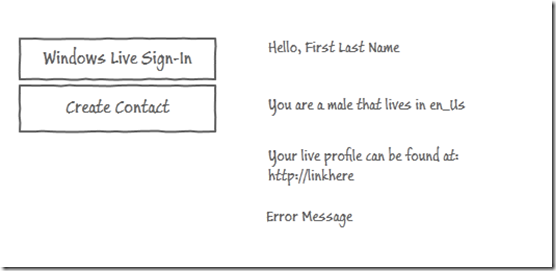
As you can see we have 2 buttons:
- Windows Live Sign-In – Provided by the Live SDK and is used to log the user into the app to retrieve Live Services information.
- Create Contact – Just a regular button that will be used to create a contact in the users address book.
On the left hand side you can see information retrieved by the Live SDK including first and last name, gender, location and live profile URL.
Double click on your MainPage.xaml and replace the existing Grid with the code snippet provided below.
1: <Grid x:Name="LayoutRoot" Background="#FF0C0C0C">
2: <Grid.ColumnDefinitions>
3: <ColumnDefinition Width="251*"/>
4: <ColumnDefinition Width="1116*"/>
5: </Grid.ColumnDefinitions>
6: <StackPanel Grid.ColumnSpan="2">
7: <live:SignInButton Name="btnLogIn" Scopes="wl.signin wl.basic wl.contacts_create"/>
8: <Button x:Name="btnCreateContact" Content="Create Contact" Click="btnCreateContact_Click" />
9: <TextBlock Name="tbName" Width="600" Height="150" FontSize="32" TextWrapping="Wrap" />
10: <TextBlock Name="tbGender" Width="600" Height="150" FontSize="32" TextWrapping="Wrap" />
11: <TextBlock Name="tbLiveProfile" Width="600" Height="150" FontSize="32" TextWrapping="Wrap" />
12: <TextBlock Name="tbError" Text="Error Message" Width="600" Height="150" FontSize="32"/>
13: </StackPanel>
14:
15: </Grid>
You will also want to make sure that your XML Namespaces for the Live SDK have been added to the page:
1: xmlns:live="using:Microsoft.Live.Controls"
Note: You will get “Invalid Markup” with the XAML provided because we are still in the developer preview stage. It is ok to proceed.
The only thing to really pay attention to here is the Scopes listed in the SignInButton. As you see we have three scopes.
- wl.signin - Single sign-in behavior. With single sign-in, users who are already signed in to Live Connect are also signed in to your website.
- wl.basic - Read access to a user's basic profile info. Also enables read access to a user's list of contacts.
- wl.contacts_create - Creation of new contacts in the user's address book.
The first two are core and the last is an extended scope. We need all three of these to complete the requirements listed in this sample application.
This will give us our user interface, now we will just need to wire up the event handlers which I am going to split up into two sections for learning purposes.
Coding Time – Basic User Information
Let’s go ahead and add in functionality to retrieve basic user information from the Live SDK.
First, add the proper namespaces and then the following code:
1: using Microsoft.Live;
2: using Microsoft.Live.Controls;
1: private LiveConnectClient liveClient;
2: private LiveConnectSession session;
3:
4: public MainPage()
5: {
6: InitializeComponent();
7: this.btnLogIn.SessionChanged += btnLogIn_OnSessionChanged;
8: }
9:
10: private void btnLogIn_OnSessionChanged(object sender, LiveConnectSessionChangedEventArgs e)
11: {
12: if (e.Session != null && e.Status == LiveConnectSessionStatus.Connected)
13: {
14: this.liveClient = new LiveConnectClient(e.Session);
15: session = e.Session;
16: this.liveClient.GetCompleted += OnGetCompleted;
17: this.liveClient.GetAsync("me", null);
18: }
19: else
20: {
21: this.liveClient = null;
22: }
23: }
24:
25: private void OnGetCompleted(object sender, LiveOperationCompletedEventArgs e)
26: {
27: if (e.Error == null)
28: {
29: dynamic result = e.Result;
30: this.tbName.Text = "Hello, " + result.first_name + " " + result.last_name;
31: this.tbGender.Text = "You are a " + result.gender + " that lives in " + result.locale + ".";
32: this.tbLiveProfile.Text = "Your Live Profile can be found at: " + result.link;
33: }
34: else
35: {
36: this.tbError.Text = e.Error.ToString();
37: }
38: }
The first thing that you will notice is that we are creating an event hander to handle the SessionChanged event. If we can determine that the session is valid then we create a new LiveConnectClient and pass the session to our LiveConnectSession. We finish up with adding an event handler on GetCompleted which will return the current users profile information and display them in our TextBlocks. Finally, we are outputting all errors to the tbError TextBlock.
Coding Time – Adding a new contact to the users Address Book
Let’s go ahead and add in functionality to add a new contact to the users address book from the Live SDK.
1: private void btnCreateContact_Click(object sender, RoutedEventArgs e)
2: {
3: LiveConnectClient createContact = new LiveConnectClient(session);
4: var contact = new Dictionary<string, object>();
5: contact.Add("first_name", "Michael");
6: contact.Add("last_name", "Crump");
7: createContact.PostCompleted +=
8: new EventHandler<LiveOperationCompletedEventArgs>(CreateContactProperties_PostCompleted);
9: createContact.PostAsync("me/contacts", contact);
10:
11:
12: }
13:
14: void CreateContactProperties_PostCompleted(object sender, LiveOperationCompletedEventArgs e)
15: {
16: if (e.Error == null)
17: {
18: tbName.Text = "Contact" +
19: e.Result["first_name"].ToString() + " " + e.Result["last_name"].ToString() +
20: " created with ID " +
21: e.Result["id"].ToString();
22: }
23: else
24: {
25: tbError.Text = "Error calling API: " + e.Error.ToString();
26: }
27: }
In this sample, we are creating a new LiveConnectClient by passing into it the existing session. Once that is complete, we create a Dictionary and add the users first_name and last_name to the dictionary. Finally, we create a PostCompleted event handler which will return the textblock with a success or an error. The last call is PostAsync which send a POST request to the specified Uri as an asynchronous operation.
Ready for Liftoff
Go ahead and build the application and run it.
When you first hit the “Sign In” Button you will be presented with the following:
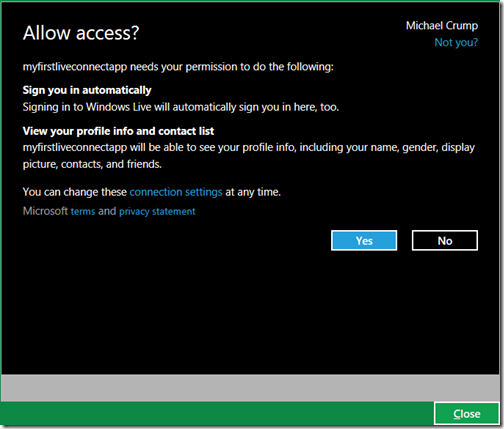
Note: After you accept this one time then you will not have to accept it again.
Notice that the application says it you allow access then it will view your profile and contact list. The application automatically knows which features it is using by the scopes defined earlier.
Select “Yes” and then click the “Sign In Button” located on the right hand side of your screen and you should see your Live profile information as long as you have filled it out in Windows Live.
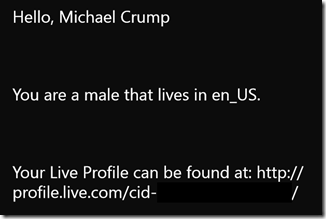
Next, click on the Create Contact button and you should see the following:

You can now sign into your Windows Live account and select “Contacts” under “Hotmail” and see the contact that you just created.
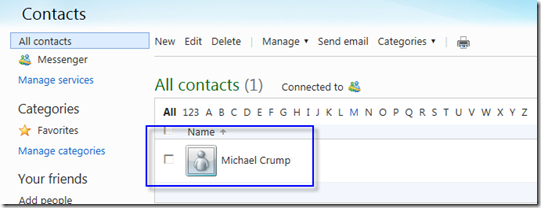
Congratulations, you have now completed your first Metro application using XAML/C# that uses the Live SDK.
Conclusion
At this point, we have seen how you would create a Windows 8 Metro application using XAML/C# that retrieves and updates information from the Live SDK. Now that you are equipped with the basics of using the Live SDK, I encourage you to explore the Live SDK further as we have barely scratched the surface. I want to thank you for reading this article and if you ever have any questions feel free to contact me on the various sources listed below. I also wanted to thank SilverlightShow for giving me the opportunity to share this information with everyone.
You can follow Michael on Twitter at mbcrump or keep up with his blog by visiting michaelcrump.net.