In this third part about communication feature improvements in Windows Phone 8, I will focus on Near Field Communication, or NFC for short, which lately has found its way into many modern smart phones across different platforms like Android, Bada or BlackBerry. On Windows Phone, NFC support has only been added in version 8; there wasn't any support for it in Windows Phone 7 neither built-in nor available to third-party developers. The most prominent use case is for payment services like the "Wallet Hub" in Windows Phone [1], however the range of uses is not limited to that, and with the available API developers can adapt the technology easily for their particular needs.
What NFC Is
Very much like Bluetooth, that we have learned about in the last part [2], Near Field Communication is a standard for short-range wireless connectivity. In contrast to Bluetooth which works in the range of up to tens of meters in optimal environments, NFC is limited to an extremely short range of just a few (typically no more than 3-5) centimeters.
NFC can be used to transfer any kind of data, but it is not only designed for communication between devices. It also supports one-way applications where active devices like NFC-enabled smart phones interact with passive (unpowered) infrastructure like RFID or NFC tags, something that you might have heard about before.
Near Field Communication requires low power and can establish connections very quickly (in less than 1/10th of a second), but its transfer speeds are slower than those of other wireless communication. An interesting use case hence is to use NFC as bootstrapping technology for other communication channels. For example, due to the low reception range unwanted communication between devices is unlikely; NFC-enabled devices therefore may allow to initiate typically more complex to set up connections like Bluetooth or Wi-Fi automatically, after a NFC connection between two peers has been established. The benefit is that the user does not need to perform multiple manual steps like enabling a connection and confirm security measurements. This is used e.g. on some Android devices to create ad-hoc Wi-Fi connections that you do not need to configure manually at all.
NFC Features in Windows Phone
In Windows Phone 8, both scenarios that I briefly outlined above are supported:
- Direct data exchange between devices using NFC connections, and
- Establishing a higher bandwidth connection like Wi-Fi or Bluetooth using NFC as initiator
To use any NFC features, you need to set up your app accordingly first, as described in the following paragraph.
NFC Setup
No matter what you intend to do, to enable NFC use in your application you need to add the required capabilities to your application's manifest file, similar to what I've described in the article on Bluetooth (networking is only required if you plan on communicating over sockets):
You can of course also specify these directly in the WMAppManifest.xml:
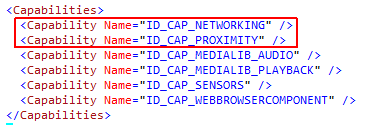
If your app cannot function in a meaningful way without NFC support, you can also enforce this on the "Requirements" tab of the WMAppManifest.xml editor. Selecting this option will prevent devices without NFC support from installing your app completely:
This of course also will simply be translated into an entry in the Xml:
Once you have configured your app for NFC use, the fundamental class involved in anything with NFC is "ProximityDevice" [3] in the Windows.Networking.Proximity namespace [4]. It offers events that notify you about the presence of other devices as well as a rich set of methods to publish NFC messages and subscribe for their reception.
The first step always should be to check whether NFC is actually supported on the device your app is currently running on. This is done by checking whether the default proximity device is null:
1: var proximityDevice = ProximityDevice.GetDefault();
2: if (proximityDevice != null)
3: {
4: // we can use NFC!
5: }
Now you can start using all the NFC features.
NFC Messages
Direct data exchange over NFC connections uses the above mentioned NFC messages. A message consists of both the message type and the actual payload to transmit. You can always define custom message types that are only meaningful to your own application, however Windows Phone also understands and supports a selection of predefined and standard message types. I'll talk a bit more about this later, after we've seen an example. To publish a message, you simply can use one of the available methods on the proximity device class. The available options include publishing string, binary and URI messages.
1: var publicationId = proximityDevice.PublishMessage(
2: "Windows.MyCustomMessageType", // message type
3: "Hallo World!", // message payload
4: MessageTransmittedHandler); // callback
5:
6: // [...]
7:
8: private void MessageTransmittedHandler(ProximityDevice sender, long messageId)
9: {
10: // the message has been transmitted
11: }
The snippet uses a custom message type of "Windows.MyCustomMessageType". The part before the dot is named "protocol" and needs to have a fixed value of "Windows" when you want to use custom messages. The latter part of course is up to you to define, as well as the payload data structure.
It's important to know that only one message at a time can be published. This means that if you want to publish a different message, you need to explicitly stop publishing first, using the corresponding "StopPublishingMessage" method [5]. This method takes the message/publication Id that is returned from the "PublishMessage" method call in the above sample. Another typical pattern also would be to stop publishing when a message has been transmitted, which is why the transmitted handler also takes the message Id argument.
The consuming side works in a similar way: once you have obtained access to the default proximity device, you can subscribe for messages. This obviously requires that you provide the same message type that is used by the publishing code.
1: proximityDevice.SubscribeForMessage("Windows.MyCustomMessageType", MessageReceivedHandler);
2:
3: // [...]
4:
5: private void MessageReceivedHandler(ProximityDevice sender, ProximityMessage message)
6: {
7: var receivedString = message.DataAsString;
8: }
Of course the "ProximityMessage" class [6] also grants access to the binary content of the message instead of the convenient string access. If you want to unsubscribe from messages, the proximity device also offers a "StopSubscribingForMessage" method.
As you can see, sending and receiving messages is pretty straight-forward and simple.
Supported Message Types
Apart from custom message types, Windows Phone also supports standard protocols and types that can be used for a greater variety of functionality, for example to transmit files or launch apps either by package id or by registered protocols (also see my article on that topic for more information [7]). The latter also allows you to launch built-in apps like the web browser and display a specific web site by publishing the corresponding NFC messages. In all these situations the user is always asked for consent due to security considerations.
A more common use case for the standard protocols is to have phones react to passive tags or NFC chips. In a way, this can be compared to e.g. using Bing Vision to read a QR code which takes you to a web site – except that you'd tap smart posters or similar accessories that contain NFC tags instead. One of the supported messages types is to actually write to tags, so you can even create the content for these tags directly from the phone.
An excellent and exhaustive article on NFC tag support in Windows Phone 8 can be found in the Nokia Developer Community Wiki [8]. It not only describes the possibilities (and limitations) of writing to tags with Windows Phone 8, but also contains a comprehensive list of supported protocols and sub types.
In addition, projects are starting to show up to help you with working with the NFC features of both Windows Phone 8 and Windows 8 in a more comfortable way. For example, the NDEF Library on CodePlex [9] gives you more control over the message details and lets you work easily with certain features like launching apps or settings pages on the phone.
Establishing Socket Connections Over NFC
As I mentioned in the beginning, another very interesting use case of NFC is to initiate higher bandwidth connections on more capable wireless connectivity technologies. Windows Phone supports exactly that by the use of the "PeerFinder" class that we've already seen in detail in my article on Bluetooth [10]. I won't go into the concepts of that class again here but rather show you how it is used to create an initial NFC connection between two devices that is then automatically "elevated" to a Bluetooth or Wi-Fi communication channel.
One key part of this procedure is the "TriggeredConnectionStateChanged" event [11] that notifies you about the different stages an NFC-initiated connection goes through until it can be used in your code. This is useful to e.g. notify users of your app when a tap was successful and they can pull away their phones again. Consuming the event also is necessary to determine which device has initiated the tap, and to obtain a reference to the "StreamSocket" instance that is used for the actual communication. This socket will already use Bluetooth or Wi-Fi (TCP/IP) as transport, depending on how you configured the PeerFinder. Take a look at this snippet for an example:
1: private void StartFindingPeer()
2: {
3: // check if the device supports NFC
4: if ((PeerFinder.SupportedDiscoveryTypes & PeerDiscoveryTypes.Triggered) == PeerDiscoveryTypes.Triggered)
5: {
6: PeerFinder.TriggeredConnectionStateChanged += TriggeredConnectionStateChanged;
7:
8: // indicate we only want a Bluetooth connection, no Wi-Fi
9: PeerFinder.AllowBluetooth = true;
10: PeerFinder.AllowInfrastructure = false;
11:
12: // go!
13: PeerFinder.Start();
14: }
15: }
16:
17: private void TriggeredConnectionStateChanged(object sender, TriggeredConnectionStateChangedEventArgs args)
18: {
19: switch (args.State)
20: {
21: case TriggeredConnectState.PeerFound:
22: // NFC tap was successful => phones can be pulled away now;
23: break;
24: case TriggeredConnectState.Connecting:
25: // this is the initiating device
26: break;
27: case TriggeredConnectState.Listening:
28: // the other device is the initiating device
29: break;
30: case TriggeredConnectState.Completed:
31: // you can use the socket to communicate!
32: var socket = args.Socket;
33: break;
34: case TriggeredConnectState.Failed:
35: case TriggeredConnectState.Canceled:
36: break;
37: }
38: }
The code shows another way of determining whether the device supports NFC in its first if-statement. Eventually you will gain access to a StreamSocket instance that you can use for communication just like with any regularly established connection.
One of the limitations on Windows Phone, again out of security considerations, is that it's not possible to automatically enable features on the phone that the user has turned off. This means that you cannot initiate a Bluetooth connection in the way shown above if Bluetooth is deactivated. Therefore, you would typically ask your users to turn on Bluetooth before tapping their phones. To make this most comfortable, you can navigate them to the corresponding settings page automatically. It's a small sacrifice of comfort in favor for more security, but requiring users to simply activate Bluetooth still is a lot better than have them pair devices and complete the normal Bluetooth setup manually.
Testing NFC
As with Bluetooth features, testing NFC is not exactly straight forward and often requires manual testing on real hardware. However, the situation is a bit better with NFC as people already have started developing tools that support testing with the built-in emulators. One of these tools is the Proximity Tapper hosted on CodePlex [12] that allows to inject NDEF messages into the emulator and also analyze the message traffic. It's not a full replacement for testing with a real phone and/or tags, but it's at least a start to make the development process a bit more efficient.
Conclusion
NFC communication is another piece in the puzzle of highly interactive and networked applications current software development is headed towards. Windows Phone's support for it is proof the platform has managed to successfully turn into an early adopter with unique characteristics, ahead of some competitors like iOS (which still has no NFC support [13]). I'm excited about what applications we will see that make use of these new opportunities.