Now that Windows 8 has been around in its final form for almost a year, developers are getting used to the new platform. Lots of paradigms that have been around for many years in the Windows environment however have changed with the new course that Microsoft is sailing with the introduction of Metro (aka Modern UI aka Windows Store) apps.
Personally, one of the most striking differences between the desktop mode and the Windows 8 environment is without a doubt the way Windows handles multiple processes. In other words, background processing and the process lifecycle of Windows 8 applications. What used to be something we didn’t really have to take into account, has now propagated itself as one of the most concept topics in Windows 8.
In this new article series, we are going to take a look at how Windows 8 manages the process lifecycle of apps and we are going to take a look at the different options we have to build applications that run in the background. In this very first article, we are going to discuss the lifecycle of Windows 8 apps itself and take a look at how Windows – and no longer the end user – is managing when applications will be running and when they won’t.
Fasten your seatbelt, as the content in these articles is quite advanced!
Process lifecycle of Windows 8 applications
So far, as end-user, in the desktop mode (or should I now say traditional Windows environment), we have always had the possibility to open up as many applications as we want. The limitation is the memory present in the machine, which will cause the machine to run slower if we open up too many apps at the same time. Many open applications also mean that the CPU is getting hammered with requests to perform work, again causing the machine to run less smooth. It’s up to that end-user to decide he closes down a few applications when this happens, freeing up memory and the CPU. The important aspect here it’s that it is the user who remains in charge of the process lifecycle.
With Windows 8, this has changed (of course, I’m referring to the Modern UI environment, in its desktop mode, this paradigm still stands). Whereas in desktop mode it’s the user who has to shut down apps, in Windows 8, Windows will now manage the process lifecycle. Windows will make sure that the app running as the main app (and optionally the app running in snapped mode) will receive as much resources as they need. The other apps don’t get access to the CPU, memory or network. They are being suspended when they are not actively being used by the user. The idea here is that the application running as the main app receives all it needs to perform great while other apps are being suspended, not using up any resources.
The direct consequence of this is that we as developers will have to write code that deals with this. For one, we’ll need to support being suspended, making sure that we can restore the application’s state later on. Also, we won’t be able to write code in the main application that simply keeps running even when the user is using another application. Luckily, there’s a whole API available, based on a system of application-level events that we can hook into to support these state transitions for the application. Let’s first start by looking at the different states an application can be in and which events can be associated with the state transitions in the process lifecycle.
The process lifecycle in Windows 8 is as follows:
- Initially, an application isn’t running. The user taps on a tile, which will launch the application. On the app-level, the launched event is firing, allowing us to build up the application. We’ll talk about the launching of the application further.
- The application is now in the running state. As long as the user keeps using the application (in other words as long as it remains the main application), the application gets full access to system resources.
- Once the user decides to start using a different application, Windows will trigger a suspension for the first application. The Suspending event will trigger, and we need to add code that saves the application state so that we can restore it later. The application is now suspended. The application remains in memory!
- From suspended state, the application can be resumed by the user. If the app was still in memory (so it’s being resumed from a suspended state), the switch to the app is immediate (it’s after all still the same application instance). Another event, the resuming event, is being raised in the application.
- If however, the user has too many applications in suspended state, Windows may decide it will start killing off a few. Suspended apps can be terminated by Windows at any time without an event being triggered (which makes sense: there’s no code running so there’s no event that can be fired in the application either). This termination is probably the most important one to design for.
I just mentioned that termination is important. The basic idea is that the user should never be bothered with the fact that the application can be terminated. For the user, the application should give the impression it has been running all the time. So when the user has unsaved data and he simply switches apps, it’s our task to save that data when the application gets in the event that we can use for this, the suspending event. That event is quite simply the last point in time we can use to save data we need to restore the application’s state later on.
If the application is being resumed and it was still in suspended state we don’t need to restore anything at all: the suspended instance was the same as the one we had left before. However, if the user switches back to an app that has been terminated by Windows, we need to restore the state that we have saved in the suspending event.
The following graphic shows the state transitions:
We can see this in action as follows:
- We tap on the Travel app. The app wasn’t running previously.
- The Travel app now becomes the main app and it gets access to all system resources.
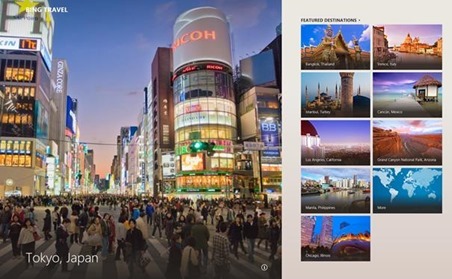
- We now open another application, let’s say we start the Music app. Now this app becomes the main running application.
- If we now take a look at the Task Manager, we can see that after a few seconds, the Travel app enters the Suspended state. The Music app is not suspended.
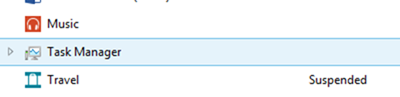
- When I go to the running applications on the left (or swipe from the left), we see that the Travel app is listed there, although it’s suspended. This means that Windows left the application’s memory image intact but we can resume it immediately.
- Should Windows now have terminated the Travel app, it won’t be showing here. However, when the user taps the Tile again in the Start screen, the app should be displaying its previous state.
Now that we have an understanding of how Windows manages the lifecycle, let’s take a look at the different events taking place in code.
In the App.xaml.cs, so at the application level, in the constructor, we can register to be notified about suspension and resuming:
public App()
{
this.InitializeComponent();
this.Suspending += OnSuspending;
this.Resuming += App_Resuming;
}
In the same class, we can write code in the OnLaunched override. The default that is added to a newly created project is as follows:
protected override void OnLaunched(LaunchActivatedEventArgs args)
{
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null)
{
// Create a Frame to act as the navigation context and navigate to the first page
rootFrame = new Frame();
if (args.PreviousExecutionState == ApplicationExecutionState.Terminated)
{
//TODO: Load state from previously suspended application
}
// Place the frame in the current Window
Window.Current.Content = rootFrame;
}
if (rootFrame.Content == null)
{
// When the navigation stack isn't restored navigate to the first page,
// configuring the new page by passing required information as a navigation
// parameter
if (!rootFrame.Navigate(typeof(MainPage), args.Arguments))
{
throw new Exception("Failed to create initial page");
}
}
// Ensure the current window is active
Window.Current.Activate();
}
There are some important things happening here. Note that the arguments (an instance of the LaunchActivatedEventArgs) has the PreviousExecutionState property. This property is an important one, since we can use it to determine if the app was terminated in a previous run or it’s a new, fresh launch. Based on this value, we can add code (see further) to restore state. Note also that the code in the Launched override will only run when the application is being launched by tapping on a tile (so not when a contract activates the application).
The default OnSuspending code contains code that uses Microsoft’s default implementation for state management, the SuspensionManager class.
private void OnSuspending(object sender, SuspendingEventArgs e)
{
var deferral = e.SuspendingOperation.GetDeferral();
//TODO: Save application state and stop any background activity
deferral.Complete();
}
Again, one very important thing that’s missing here, is the code that would run when the application is being terminated. However, there’s no override that resembles anything like OnTerminated available, which means that the only point in time we can run code that saves state is in the OnSuspending.
There are some important time considerations to make around the suspending event. First, the suspension will only trigger after around 5 seconds. This is built-in so that a quick app switch to check your mail wouldn’t trigger suspension. Secondly, when suspension does happen, your code that handles the state saving can only run for about 5 seconds as well. This means that if you would try to execute a long-running task in your OnSuspending code, your code would probably be terminated before it finishes, resulting in no state being correctly saved at all. If you use asynchronous code in the OnSuspending, you’ll need to use the deferral as shown above to avoid being suspended before the asynchronous code has finished.
One last word on Resuming. By default, the OnResuming code isn’t generated. There’s a reason for that: many apps don’t need it. In fact, the OnResuming is most often used to refresh data, for example when a Twitter app needs to refresh tweets when it’s being resumed.
Managing state
It should be clear by now that Windows 8 apps require developers to write code that manages the state of the application. Things like saving the state when the application is suspended is something extra we have to do. The ultimate goal is as said: giving the user the idea that the application has been running all the time. Should the application happen to be terminated in the meantime, the application’s state must be restored. Figuring out if we were terminated or not, can be done using the PreviousExecutionState property. One thing to note here also is that we may not have to manage state at all, it all depends a bit on the application scenario. A Twitter client may not need to save state for example. However, a data-entry application probably needs to save state for sure, since the user may have already entered quite a lot of information when he switches apps. If Windows should decide to terminate the suspended application, we need to be able to restore it. So it all depends a bit on the case of your app.
That being said, how can we then save the state? What options do we have? Well, in fact, we have quite a few options available. We can use the local storage (Application Data API), where we can save files or settings. We can also use the roaming storage (again part of the Application Data API), so that saved state would automatically be synced over the cloud. We could also use a database (SQLite) or even store the state information in a database behind a service (although in this case, we need to pay attention for the 5 second time limitation!).
Another option is using the SuspensionManager, which is a helper class, included in some Visual Studio 2012 templates for Windows 8. This class does a few things out-of-the-box for us. It has a dictionary on-board that we can use to save and restore values with. Automatically, when we then call the SaveAsync() method of the SuspensionManager class, this dictionary is persisted for us for retrieval when we need to restore data.
private async void OnSuspending(object sender, SuspendingEventArgs e)
{
var deferral = e.SuspendingOperation.GetDeferral();
await SuspensionManager.SaveAsync();
deferral.Complete();
}
However, the SuspensionManager does more than this. It also allows to keep track of the navigation history, which is also part of the state of an application. Remember that the end user is ignorant to the application being terminated and therefore, all data to restore the application in its previous state should be persisted. That includes navigation history.
The SuspensionManager can do that for us by using one simple call: RegisterFrame. In the launching of the application, we need to register the frame of the application with the SuspensionManager and that’s it. It will now keep track of the navigation history and when we call on the SaveAsync(), the history will be persisted for us as well.
protected override async void OnLaunched(LaunchActivatedEventArgs args)
{
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null)
{
// Create a Frame to act as the navigation context and navigate to the first page
rootFrame = new Frame();
//Associate the frame with a SuspensionManager key
SuspensionManager.RegisterFrame(rootFrame, "AppFrame");
...
Summary
In this first part of this article series on background processing in Windows 8, we’ve discussed how Windows 8 apps run, which is a vital part for understanding how we can create code that runs in the background in Windows 8.
About the author
Gill Cleeren is Microsoft Regional Director, Silverlight MVP, Pluralsight trainer and Telerik MVP. He lives in Belgium where he works as .NET architect at Ordina. Gill has given many sessions, webcasts and trainings on new as well as existing technologies, such as Silverlight, ASP.NET and WPF at conferences including TechEd, TechDays, DevDays, NDC Oslo, SQL Server Saturday Switserland, Silverlight Roadshow in Sweden, Telerik RoadShow UK… Gill has written 2 books: “Silverlight 4 Data and Services Cookbook” and Silverlight 5 Data and Services Cookbook and is author of many articles for magazines and websites. You can find his blog at www.snowball.be. Twitter: @gillcleeren