Welcome to part 4 in this series on background processing in Windows 8. So far, we’ve covered the lifecycle of applications in Windows 8, regular background tasks and how we can “upgrade” an application to become a lock screen app. That last item there was the focus of part 3. But we finished that article with a cliff hanger… We had seen how we can allow an application to post badge updates on the lock screen. But we had done so from the foreground application. However, this article series is all about background processing… In this 4th part, we are going to extend the lock screen example so it will now be able to send updates from a background process to the lock screen.
The Push Notification Trigger
Remember how we saw in part 3 that a specific API became available for the application when the app was allowed on the lock screen? Basically, when doing so, the application can use 3 extra triggers: the Push Notification Trigger, the Network Trigger and the Time Trigger. We’ll start with the first one, the Push Notification Trigger.
We’ve already covered push notifications, so you may be thinking: what do these push notifications have to do with lock screen apps? When using PN regularly, the server will send to WNS (Windows Notification Service) a template XML message. This message contains either a tile, badge or toast string, formatted according to a specific template. The reason for this was that the incoming message on the client machine was received by the Client Notification Platform, which can only read out the templated XML strings.
Using the Push Notification Trigger in our apps allows us to create a background process that will be called when a message is coming in. In this case, we call these messages Raw notifications. Indeed, as the name implies, the message is “raw”, meaning it can be just any string. The message is sent from WNS again, triggered by a server-side system, but instead of being XML, it can be just any text message. When this string arrives on the client, the associated background task is triggered (hence the name Push Notification Trigger) and the message is passed in to the background task. This task can then do whatever it wants with this string.
Microsoft recommends using the Push Notification Trigger when possible if you need to have an open connection to a service (so for scenarios such as a Skype client). The main reason is power efficiency: all apps will be using the same open channel connection instead of creating their own (and therefore adding to the load on the system). So effectively, the Push Notification trigger is one way of creating an application that can run code when triggered from the server-side. The only requirement is of course that the user has to put the app on the lock screen, otherwise the Push Notification Trigger won’t be available to use.
Let’s take a look at extending the application to use this trigger now.
Using the Push Notification Trigger
Since we are going to create a background task that updates the badge on the lock screen upon receiving a raw push notification, we should first create this task. This will be a separate project, just like the regular background task.
In there, we again have a class that implements the IBackgroundTask interface and implements the Run method. In the code below, we are getting the raw string message using the passed-in IBackgroundTaskInstance: the TriggerDetails property needs to be cast to a RawNotification and that has a Content property. In there, we find the raw string.
1: public void Run(IBackgroundTaskInstance taskInstance)
2: {
3: RawNotification notification =
4: (RawNotification)taskInstance.TriggerDetails;
5: ApplicationDataContainer container =
6: ApplicationData.Current.LocalSettings;
7: container.Values["RawMessage"] = notification.Content.ToString();
8: }
In the application manifest, we of course need to register the background task. Here we need to indicate that we’ll be using a Push Notification Trigger and the entry point needs to be set to the class containing the background task code (so in the separate project).
Next, we need to try to get the application on the lock screen. The code for this is similar to what we saw in Part 3. However, once we are allowed on the lock screen, we need to first get hold of the ChannelUri (the unique identification for the app on the device for WNS). Once we have this value, we can send it to the server-side so it can store this value. This process is identical to what we did earlier when using WNS regularly.
1: BackgroundAccessStatus backgroundAccessStatus = BackgroundExecutionManager.GetAccessStatus();
2:
3: if (backgroundAccessStatus == BackgroundAccessStatus.Unspecified)
4: backgroundAccessStatus = await BackgroundExecutionManager.RequestAccessAsync();
5:
6: if (backgroundAccessStatus == BackgroundAccessStatus.AllowedMayUseActiveRealTimeConnectivity ||
7: backgroundAccessStatus == BackgroundAccessStatus.AllowedWithAlwaysOnRealTimeConnectivity)
8: {
9: MailService.MailServiceClient client = new MailService.MailServiceClient();
10: var mails = await client.GetUnreadMailsForCustomerAsync
11: (new Guid("{E7BD05AC-DD3C-4904-A4A3-1E7C4C9421D0}"));
12:
13: int count = mails.Count;
14:
15: var channelUri = await Windows.Networking.PushNotifications
16: .PushNotificationChannelManager.CreatePushNotificationChannelForApplicationAsync();
17:
18: ...
19:
20: RegisterBackgroundTask();
21:
22: ReadRawMessageFromSettings();
23: }
In the RegisterBackgroundTask method, we are going to use the PushNotificationTrigger to register our task with the system. We can see this in the code below.
1: private void RegisterBackgroundTask()
2: {
3: PushNotificationTrigger pushNotificationTrigger = new PushNotificationTrigger();
4:
5: var task = BackgroundTaskHelper.RegisterBackgroundTask("Tasks.MailTask",
6: "MailBackgroundTask",
7: pushNotificationTrigger,
8: null);
9: StatusTextBlock.Text = "Task registered";
10: task.Completed += task_Completed;
11:
12: }
When we run the code now, we can see that the string sent by our service to WNS arrives in the background task (which gets triggered). We can use this string in whatever way we want. For example, this string can include a JSON-serialized object.
The Network Trigger
Using the Push Notification trigger requires that we have control over the server-side, since we need to be able to configure the server-side code so it will start sending raw messages to the client. If this isn’t possible, you won’t be able to use this type of trigger. If you however require to have an open connection to your application, you can use the Network Trigger.
Using this trigger results in having a “real” open socket connection, only for use for your application. So in terms of efficiency, this is less favorable than re-using the same connection between several apps with the push notification trigger.
Using this trigger is quite complex. If you’re interested in learning more about this, I’ve created a Pluralsight course on background processing where I do a deep-dive in using the Network Trigger. If you have a subscription, you can watch the video here: http://pluralsight.com/training/Courses/TableOfContents/win8-bgproc
The Time Trigger
To finish off this article, we are going to take a look at the Time Trigger. So far, we haven’t seen a way to run code on a regular/time-based interval. Indeed, it’s out-of-the-box not possible to create a regular background task that runs every now and then. This may sound weird (it IS weird in my opinion) but that’s how it’s created by Microsoft at this point.
The Time Trigger however does come close in allowing us to run code at specific intervals. To be able to use this type of trigger, the application again needs to be on the lock screen. Another limitation of this trigger is that the smallest interval is 15 minutes. This may work for some scenarios but it won’t for others. For example, we could use the Time Trigger to check a service for new mail every so often. If the user wants a smaller interval, well… that isn’t possible with the TimeTrigger.
Assume we want to add support for this trigger as well. Code-wise, not a lot is changing. The code is still in a separate project and still has the implementation in the Run method. However, the registration of the background task in the main application is different: we need to use the TimeTrigger here.
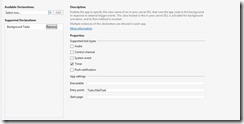
Note that again here, the entry point is set to the background task code.
In code, to register the background task with a trigger, we are now going to use the TimeTrigger instead of the Push Notification Trigger. The TimeTrigger class has 2 parameters. The first one is of course the interval, which can’t be smaller than 15 minutes. The second parameter, oneShot, allows us to indicate if this task should trigger once or continue to fire after the interval has passed. All this can be seen in the code below.
1: private void RegisterBackgroundTask()
2: {
3: TimeTrigger timeTrigger = new TimeTrigger(15, false);
4:
5: var task =
6: BackgroundTaskHelper.RegisterBackgroundTask
7: ("Tasks.MailTask",
8: "MailBackgroundTask",
9: timeTrigger,
10: null);
11:
12: task.Completed += task_Completed;
13: StatusTextBlock.Text += "Task registered\n";
14: }
After the interval has passed, the task will execute.
Summary
In this fourth part of this article series on background processing, we looked at how we can update or display information on the lock screen from background tasks. To do so, we used the Push Notification Trigger and the TimeTrigger. The Network trigger hasn’t been covered in this article.
In the next part, we’ll look at background transfers. Stay tuned!
About the author
Gill Cleeren is Microsoft Regional Director, Silverlight MVP, Pluralsight trainer and Telerik MVP. He lives in Belgium where he works as .NET architect at Ordina. Gill has given many sessions, webcasts and trainings on new as well as existing technologies, such as Silverlight, ASP.NET and WPF at conferences including TechEd, TechDays, DevDays, NDC Oslo, SQL Server Saturday Switserland, Silverlight Roadshow in Sweden, Telerik RoadShow UK… Gill has written 2 books: “Silverlight 4 Data and Services Cookbook” and Silverlight 5 Data and Services Cookbook and is author of many articles for magazines and websites. You can find his blog at www.snowball.be. Twitter: @gillcleeren