Since the first beta release of Silverlight 2 there was a lot of talk about controls like a TreeView, auto complete TextBox, WrapPanel, DockPanel etc, and because of the high demand some custom controls were created. Last week the Silverlight Toolkit was released and introduced the so long awaited controls. In this article we'll take a closer look to one of them - the DockPanel.
Introduction
The DockPanel is a layout control, like the StackPanel and the Grid, but in some cases is far more powerful than them. The idea of the control is that you can "dock" its child controls in all of the four directions - top, bottom, left, right (compared to the StackPanel, which allows only two directions - top->bottom and left->right). That gives us pretty much freedom when designing our application's UI. Freedom in sense that the count of the nested controls will be less, which means less code and more simple structure of the XAML, or you don't have to bother to define the structure of a Grid first.
Here are some basic guidelines about the behavior of the control ( we'll take a closer look at some of them later on in the article):
- You can align the child controls to the top, bottom, left or right. The default alignment is to left.
- The child controls take the place that is left after placing the previous child controls.
- You can fill the rest of the place with the last child control. The DockPanel does this by default.
Getting Started
To start using the DockPanel you must first download the Silverlight Toolkit (you can find it here) and add a reference to the Microsoft.Windows.Controls.dll in your Silverlight project. After that we just declare the namespace in our XAML and define our control.
<UserControl x:Class="SilverlightTools.Page"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:toolkit="clr-namespace:Microsoft.Windows.Controls;assembly=Microsoft.Windows.Controls"
Width="400" Height="300">
<toolkit:DockPanel x:Name="LayoutRoot">
</toolkit:DockPanel>
</UserControl>
Adding some controls
In order to concentrate on the basics of the control and its behavior I will use some simple controls, like Recatngles, in my examples.
To add a control to our DockPanel we must place it inside its tag. By default it will be aligned left:
<toolkit:DockPanel Background="Gray" LastChildFill="False">
<Rectangle Fill="Red"
Width="75"
VerticalAlignment="Stretch"
Style="{StaticResource Rectangle}">
</Rectangle>
</toolkit:DockPanel>
If we want to align it to a different side of the panel, then we use the AttachedProperty Dock of the DockPanel:
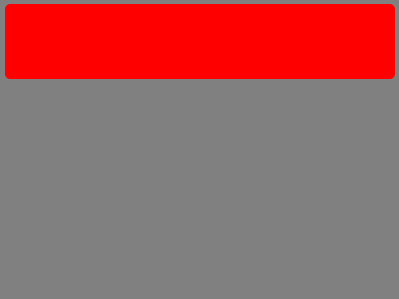
<toolkit:DockPanel Background="Gray" LastChildFill="False">
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red"
Height="75"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}">
</Rectangle>
</toolkit:DockPanel>
Maybe you're wondering why I set the Vertical- and HorizontalAlignment properties to Stretch? I do that so you can see how much place is allocated for the controls. Take a look at the images - depending on the value of the Dock property the control allocates a whole column/row with width/height depending on the dimensions of the child control and the value of Dock property. So for example if you set the Width to a value (75 for example) and remove the HorizontalAlignment property in the "Top"-oriented example, you'll see a rectangle centered in the top of our DockPanel. Note: The space left on both sides of the rectangle can't be used for other controls.
Filling the rest of the panel
The DockPanel control allows you to fill the place that's left with the last child control. This behavior is controlled by the LastChildFill property. It's default value is True. Here it is:
<toolkit:DockPanel Background="Gray" LastChildFill="True">
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red"
Height="75"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}">
</Rectangle>
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red"
Style="{StaticResource Rectangle}">
</Rectangle>
</toolkit:DockPanel>
Note that I haven't sized the last child control. If you want the DockPanel to fill the left place with it, you don't need to set the width and the height of the control, otherwise the control will be shown in its size.
Some basic Scenarios
A simple WebSite
In this scenario we need the content to take the rest of the place. It's hard to accomplish this using a StackPanel, but it can be done with the Grid control also. On the Toolkit's demo page you can find a simple text editor that implements the same scenario.
<toolkit:DockPanel Background="Gray" LastChildFill="True">
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red" Height="75"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}" />
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red" Style="{StaticResource Rectangle}"
HorizontalAlignment="Stretch"
Height="30" />
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red"
Style="{StaticResource Rectangle}" />
</toolkit:DockPanel>
Spiral
I can't imagine doing this in with a Grid control or a StackPanel control.
<toolkit:DockPanel Background="Gray" LastChildFill="False">
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red" Height="50"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}" />
<Rectangle toolkit:DockPanel.Dock="Left"
Fill="Red" Style="{StaticResource Rectangle}"
VerticalAlignment="Stretch"
Width="50" />
<Rectangle toolkit:DockPanel.Dock="Bottom"
Fill="Red"
Style="{StaticResource Rectangle}"
Height="50"
HorizontalAlignment="Stretch" />
<Rectangle toolkit:DockPanel.Dock="Right"
Fill="Red" Style="{StaticResource Rectangle}"
VerticalAlignment="Stretch"
Width="50" />
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red" Height="50"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}" />
<Rectangle toolkit:DockPanel.Dock="Left"
Fill="Red" Style="{StaticResource Rectangle}"
VerticalAlignment="Stretch"
Width="50" />
<Rectangle toolkit:DockPanel.Dock="Bottom"
Fill="Red"
Style="{StaticResource Rectangle}"
Height="50"
HorizontalAlignment="Stretch" />
<Rectangle toolkit:DockPanel.Dock="Right"
Fill="Red" Style="{StaticResource Rectangle}"
VerticalAlignment="Stretch"
Width="50" />
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red" Height="50"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}" />
</toolkit:DockPanel>
Out of space
In some scenarios when you use a fixed values for the width and the height of your child controls, you can come into a case where you have not enough space left in the DockPanel to display your control and it gets clipped. In this example the Green rectangle has height of 150px, but the place left is smaller than that.
Note: This kind of clipping can also be used on purpose.
<toolkit:DockPanel Background="Gray" LastChildFill="False" Width="400" Height="300">
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Red" Height="75"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}" />
<Rectangle toolkit:DockPanel.Dock="Bottom"
Fill="Red" Style="{StaticResource Rectangle}"
HorizontalAlignment="Stretch"
Height="75" />
<Rectangle toolkit:DockPanel.Dock="Left"
Fill="Red"
VerticalAlignment="Stretch"
Width="50"
Style="{StaticResource Rectangle}" />
<Rectangle toolkit:DockPanel.Dock="Right"
Fill="Red"
VerticalAlignment="Stretch"
Width="50"
Style="{StaticResource Rectangle}" />
<Rectangle toolkit:DockPanel.Dock="Top"
Fill="Green" Height="150"
HorizontalAlignment="Stretch"
Style="{StaticResource Rectangle}" />
</toolkit:DockPanel>
Conclusion
These were some basic guidelines about the DockPanel control, that will help you get started with it. Be sure that it has a lot more to give you than these simple examples, so don't be afraid to experiment a bit and I'm sure that after some time you'll find far more attractive than the Grid and the StackPanel in some scenarios!